No Shit Talks. Let’s begin.
Step 1: Download the snapshot builds
Download the macos-clang-arm64.tar.gz
file here https://artifacts.sfml-dev.org/by-branch/master/ and unzip it. You’ll see an ugly folder name, that’s okay.
Step 2: Copy files
cd
into that ugly-named folder and run the following commands.
$ sudo cp -r *.framework /Library/Frameworks
$ sudo cp -r ./lib/*.framework /Library/Frameworks
$ sudo cp -r ./lib/*.dylib /usr/local/lib
$ sudo cp -r ./include/SFML /usr/local/include
$ sudo chown -R <you username>:staff /Library/Frameworks
$ sudo chown -R <you username>:staff /usr/local/lib
$ sudo chown -R <you username>:staff /usr/local/include
$ sudo chmod g+rwx -R /Library/Frameworks
$ sudo chmod g+rwx -R /usr/local/lib
$ sudo chmod g+rwx -R /usr/local/include
You’ll see an Operation not Permitted
refers to the iTunes related one. Ignore it.
Remember to change <your username>
, including the two angle brackets.
Here are the folders I have in /Library/Frameworks
, /usr/local/lib
, and /usr/local/include
, respectively:

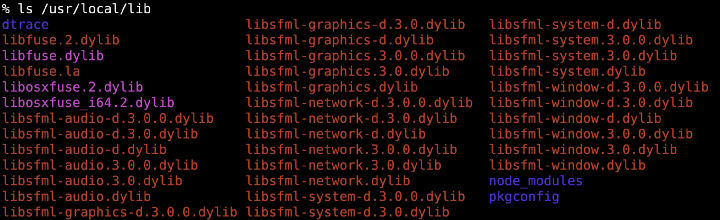

Step 3: Test Scripts
Create a main.cpp
file and add the following code. It’s a little different from the examples you see in the official documents.
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(sf::Vector2u(200, 200)), "SFML works!");
sf::CircleShape shape(100.f);
shape.setFillColor(sf::Color::Green);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed) {
window.close();
}
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
Note the sf::Vector2u
. Discussion here: https://stackoverflow.com/questions/72426626/trouble-creating-an-sfml-window. Source code: https://github.com/SFML/SFML/blob/master/include/SFML/Window/VideoMode.hpp#L62
Then run
$ g++ -std=c++17 -c main.cpp -I/usr/local/include
$ g++ main.o -o sfml -lsfml-network -lsfml-audio -lsfml-graphics -lsfml-window -lsfml-system
If you see the same window, here we go.
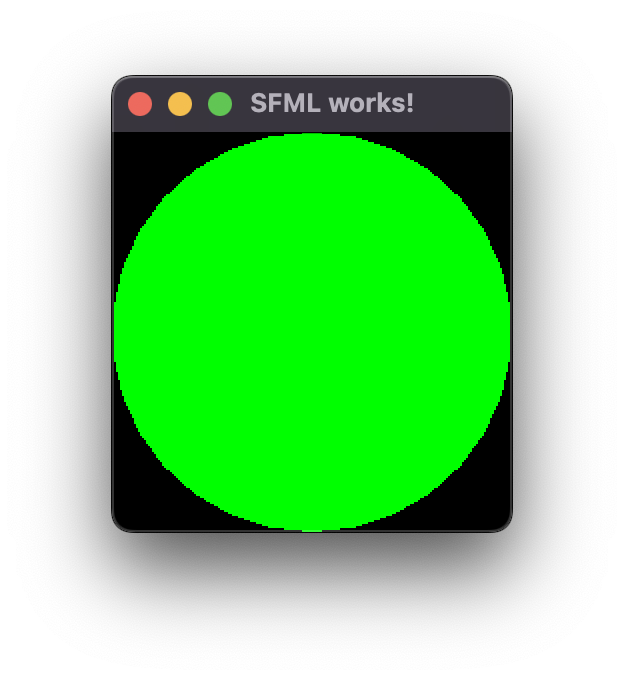
References
https://en.sfml-dev.org/forums/index.php?topic=28484.0
https://artifacts.sfml-dev.org/by-branch/master/
https://www.sfml-dev.org/tutorials/2.5/start-osx.php